Integer Overflows and Underflows
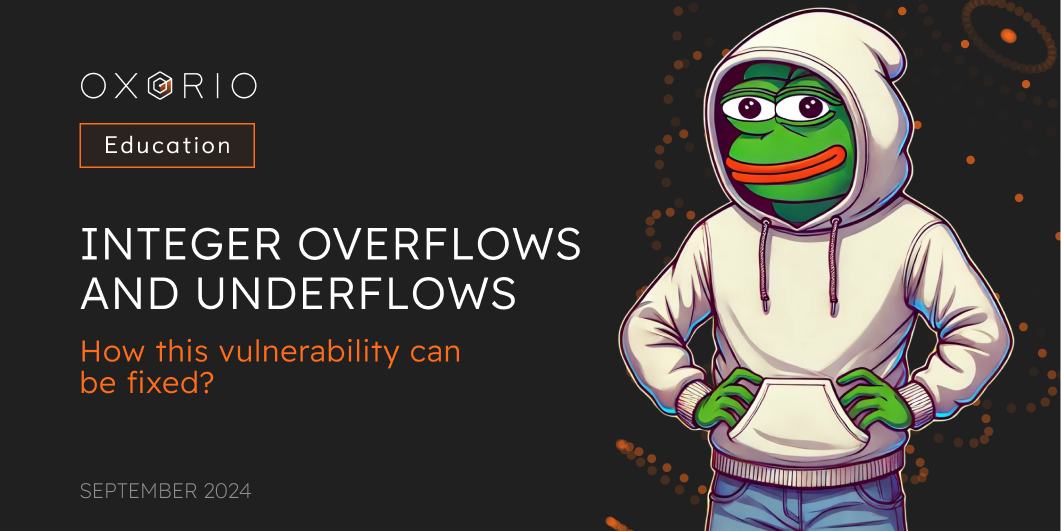
Integer overflow and underflow are common issues in programming, including Solidity.
Arithmetic operations can result to these issues when the result exceeds the representable range of numbers. This often leads to unpredictable contract behavior and poses potential security risks.
In Details
The Ethereum Virtual Machine (EVM) imposes size limitations on integer data types. Each type has a fixed range of values. For instance, a variable of type uint8 can only hold integer values from 0 to 255, inclusive. Any attempt to store a value greater than 255 will result in an overflow error. Similarly, subtracting 1 from 0 in a uint8
variable will yield 255 due to a phenomenon known as underflow.
When performing arithmetic operations, if the result falls outside the valid range for the given data type, an overflow or underflow occurs. For signed integers, which can represent both positive and negative values, the situation is slightly different.
If, for example, 1 is subtracted from a variable of type int8
with a value of -128, the result will be 127. This is due to the specific representation of negative numbers in binary code and the cyclic nature of the value range for signed types.
Let’s Take a Practical Example
Integer Overflow Example
In this example, the increment
function adds amountToAdd
to the `currentValue` variable. However, if `currentValue` is already close to the maximum value of uint256
, adding amountToAdd
might cause an overflow.
pragma solidity ^0.8.0;
contract OverflowExample {
uint256 public currentValue;
function increment(uint256 amountToAdd) public {
currentValue += amountToAdd;
}
}
Integer Underflow Example
Similarly, in this example, the subtract
function subtracts amountToSubtract
from the currentValue
variable. If currentValue
is close to zero, subtracting amountToSubtract
might cause an underflow.
pragma solidity ^0.8.0;
contract UnderflowExample {
uint256 public currentValue;
function subtract(uint256 amountToSubtract) public {
currentValue -= amountToSubtract;
}
}
Victims of Attack
Let’s take a look at some of the victims who have been affected by this attack.
- Hyperdrive
- https://solodit.xyz/issues/addliquidity-can-be-griefed-spearbit-none-hyperdrive-june-2023-pdf
- Koii Network
- Ionprotocol
- BendDAO
- Fjord
Mitigating Risks
A common solution to this problem is OpenZeppelin’s SafeMath library, which provides a set of functions for safe mathematical operations.
The main functions in SafeMath are:
- add(uint256 a, uint256 b): Returns the sum of two unsigned integers, reverting on overflow.
- sub(uint256 a, uint256 b): Returns the difference of two unsigned integers, reverting on underflow (if the result is negative).
- mul(uint256 a, uint256 b): Returns the product of two unsigned integers, reverting on overflow.
- div(uint256 a, uint256 b): Returns the integer division of two unsigned integers, reverting on division by zero.
- mod(uint256 a, uint256 b): Returns the modulo of two unsigned integers, reverting on division by zero.
Since Solidity version 0.8.0, built-in checks for overflow and underflow have been added directly to the language, making the use of SafeMath less necessary. However, the library is still available for use and may be beneficial in projects that use older versions of Solidity or for ensuring compatibility and ease of migration.
How Oxorio Can Help?
Integer overflow and underflow remain prevalent issues in programming languages like Solidity, posing significant security risks and leading to unpredictable contract behavior. These vulnerabilities occur when arithmetic operations exceed the representable range of numbers, potentially allowing attackers to exploit contracts by manipulating numerical values beyond expected limits.
Understanding and mitigating these risks is crucial for the integrity and success of your blockchain project. Here’s how OXORIO can help:
- Expert Smart Contract Audits
- Secure Coding Guidance
- Customized Solutions
- Ongoing Support and Updates
By partnering with OXORIO, you’re not just fixing a vulnerability — you’re proactively strengthening your project’s foundation. We help you build trust with your users and investors by ensuring that your smart contracts are secure, reliable, and resilient against common pitfalls like integer overflows and underflows.
Contents
YOU MAY ALSO LIKE
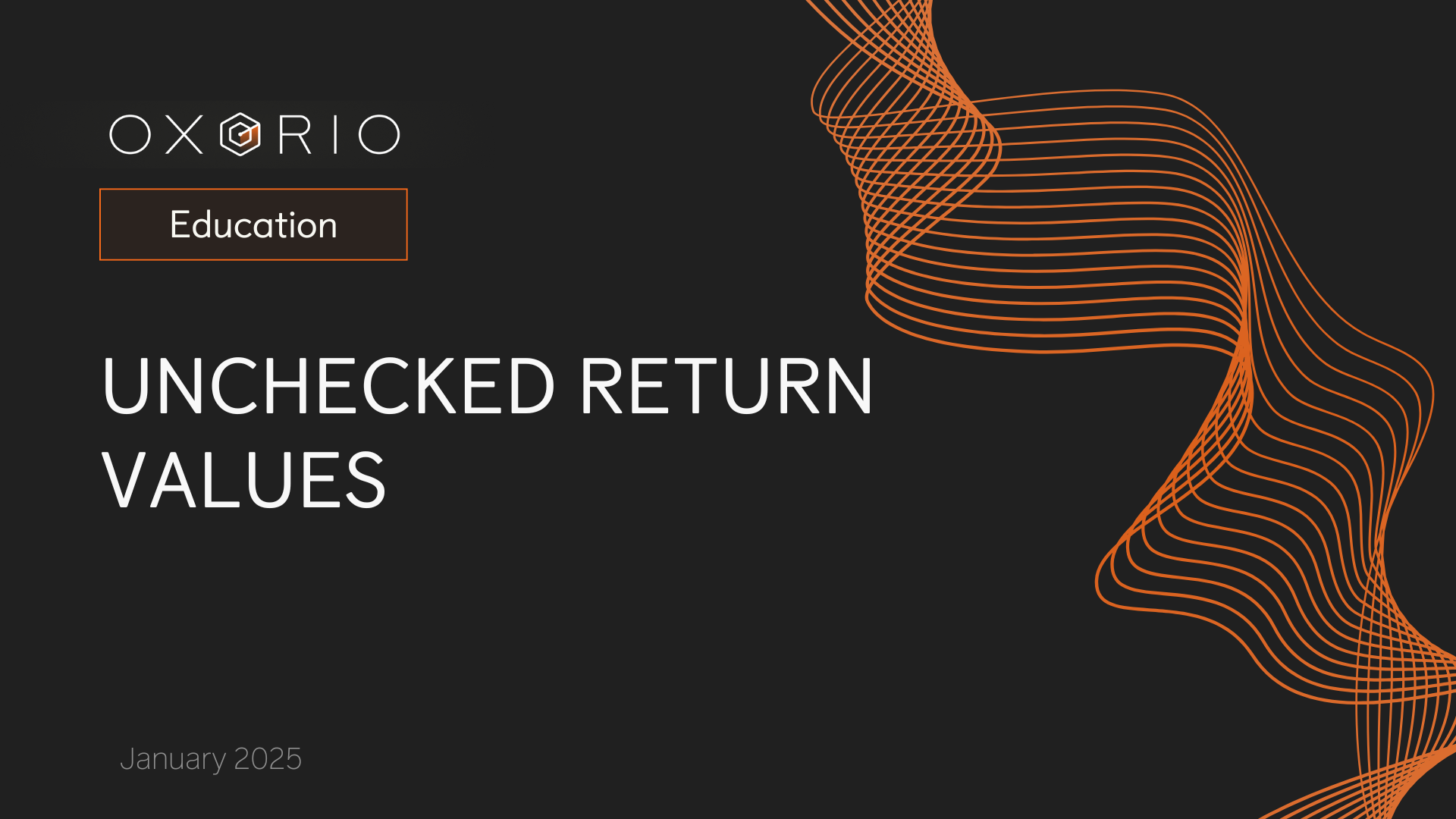
Unchecked Return Values
Education
Discover the critical risks of unchecked return values in smart contracts and how they can lead to catastrophic vulnerabilities. Learn best practices for secure coding, effective mitigation strategies, and real-world examples of exploited weaknesses.
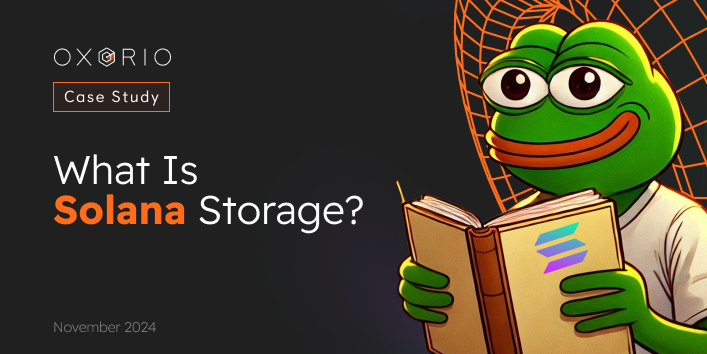
What is Solana Storage?
Education
Discover Solana's unique approach to blockchain storage with an in-depth exploration of its Programs and Accounts model.
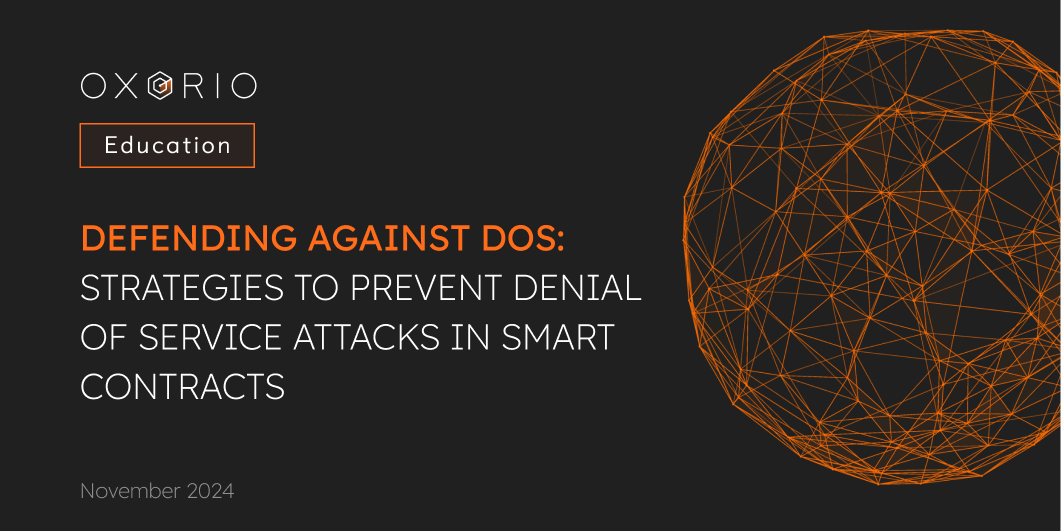
Defending Against DoS: Strategies to Prevent Denial of Service Attacks in Smart Contracts
Education
Explore how DoS attacks like Unexpected Reverts, Block Gas Limits, and Block Stuffing disrupt Solidity smart contracts. Learn security methods to safeguard your blockchain projects.
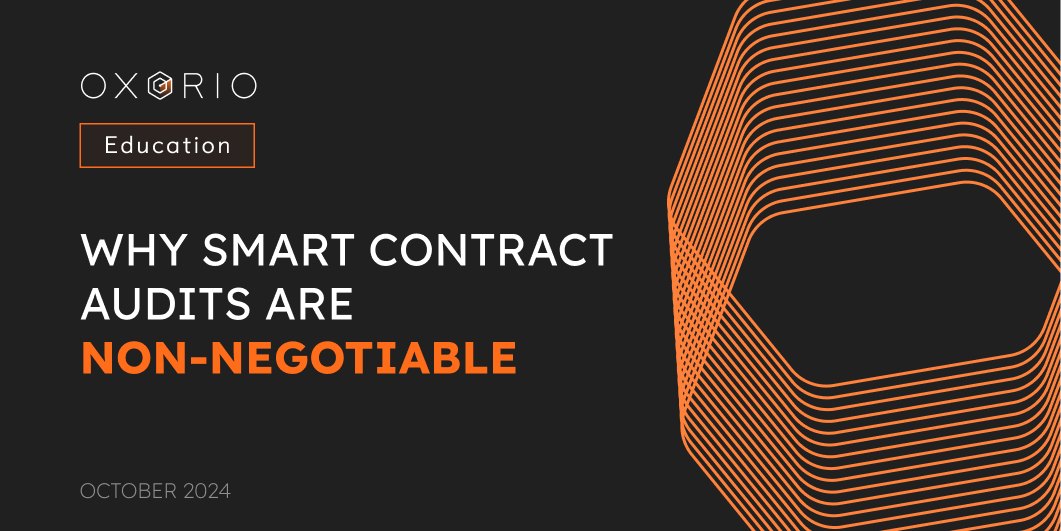
Why Smart Contract Audits are Non-Negotiable
Education
Unlock Web3's full potential by securing your smart contracts. Learn why audits are essential, explore real-world risks, and see how Oxor.io fortifies your blockchain projects against threats.
Have a question?
Stay Connected with OXORIO
We're here to help and guide you through any inquiries you might have about blockchain security and audits.